In the world of programming, particularly in Java, understanding the difference between System.out.print and return is crucial for writing functional, readable, and efficient code. Both are frequently used tools for handling values, but they serve completely different purposes. A clear understanding of each can help developers better structure their applications and debug their code more effectively.
System.out.print and return are both fundamental operations in Java, but while they may look similar when used in a beginner’s context, they possess very different behaviors and functions. Let’s delve deeper into what each of them is, how they differ, and where each should be used in a program.
System.out.print: Output to Console
System.out.print() is a method in Java used to display text on the standard output stream, typically the console. When a developer wants to show a value to the user or debug what’s going on inside the program, they might use System.out.print or System.out.println.
For example:
System.out.print("Hello, World!");
This line will print “Hello, World!” to the console but will not impact the program’s logic or output values back to the calling function.
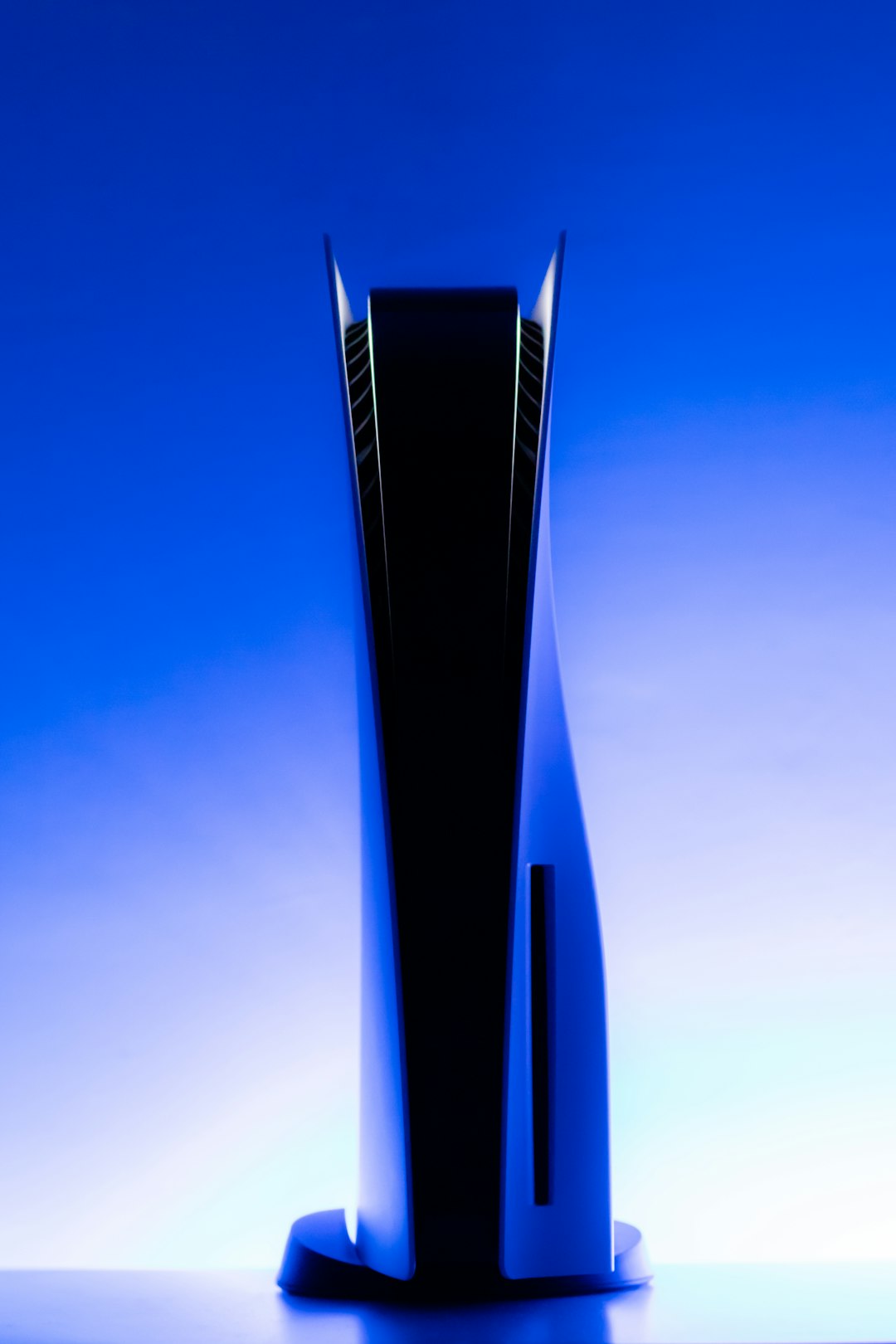
Key Traits of System.out.print:
- Used for displaying messages or values to the console
- Primarily aids in debugging or providing user interaction
- Does not return a value to any function or modify program flow
Simply put, System.out.print is for output only. It has no effect on how data moves or is handled within the program logic.
Return: Sending Data Back from a Method
In contrast, the return statement is used to exit a method and optionally send a value back to the calling code. This is essential for methods that compute results that will later be used elsewhere in the program.
Example:
public int add(int a, int b) {
return a + b;
}
Here, the method add computes the sum of two integers and returns the result wherever it was called. Unlike System.out.print, the return value influences the program’s functionality and can be stored, passed, or further manipulated.
Key Traits of return:
- Used to return data to the caller method
- Can terminate the method execution
- Plays a crucial role in program logic and flow
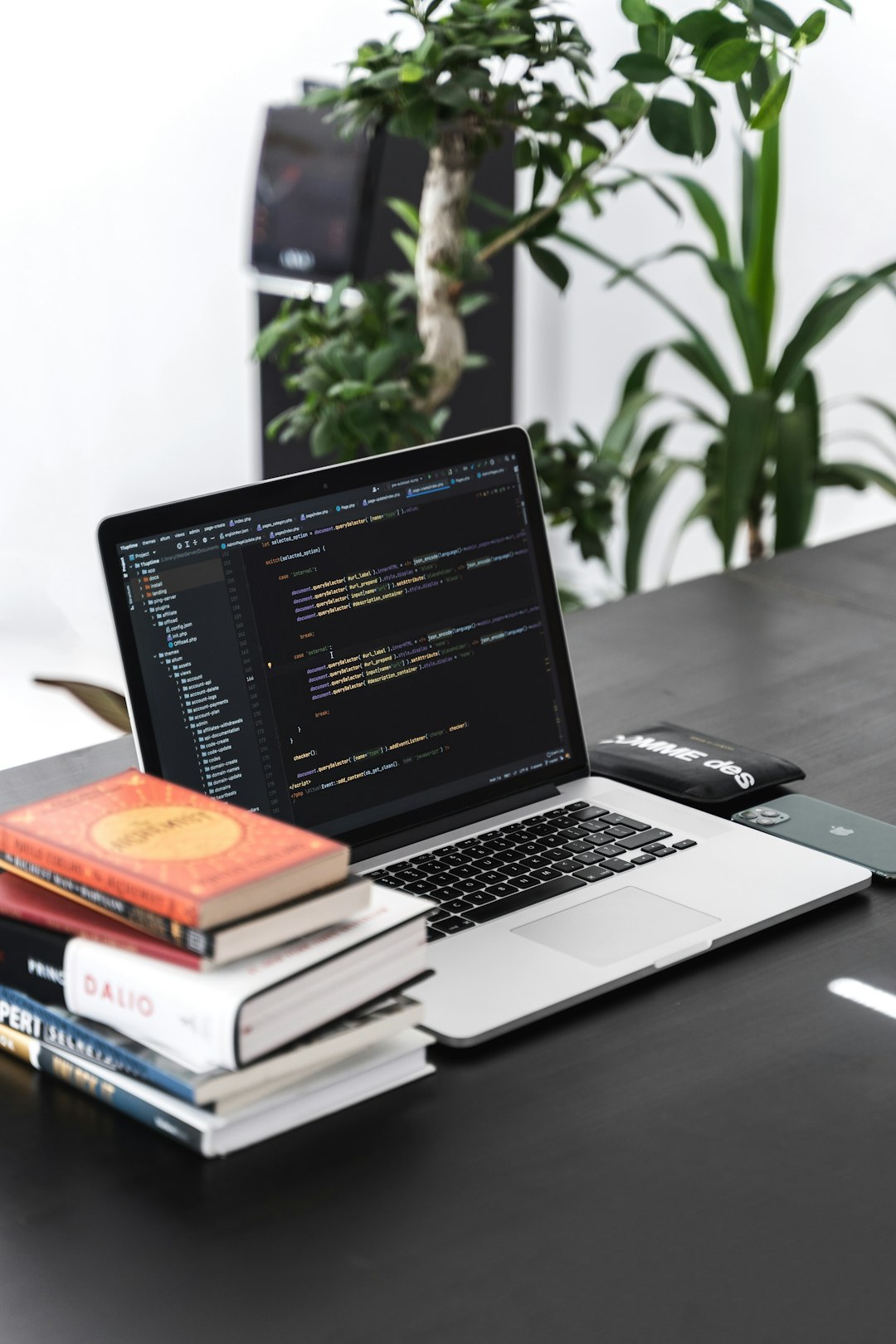
Main Differences at a Glance
- Visibility: System.out.print displays values on the console; return sends values back to a method’s caller.
- Execution Flow: Return stops the method execution and exits to the caller; System.out.print does not alter flow.
- Use Case: Use System.out.print for diagnostics and UI; use return for program decisions and logic flow.
A common mistake among beginners is using System.out.print expecting that the value printed will be available later in the program logic. However, only the return keyword will hand the data to another part of the application where it can be manipulated or stored.
When to Use Which?
Use System.out.print when:
- You want to inform the user or developer about the program’s status
- You’re debugging values to understand application behavior
Use return when:
- The result of a method needs to be reused or transferred
- The method must evaluate and provide data for a condition or computation
Understanding the distinction helps avoid pitfalls such as “Why isn’t my output being used?” or “Why is my method returning nothing?” In practice, they can also be used together: a method may return a value and before doing so, System.out.print it for logging purposes.
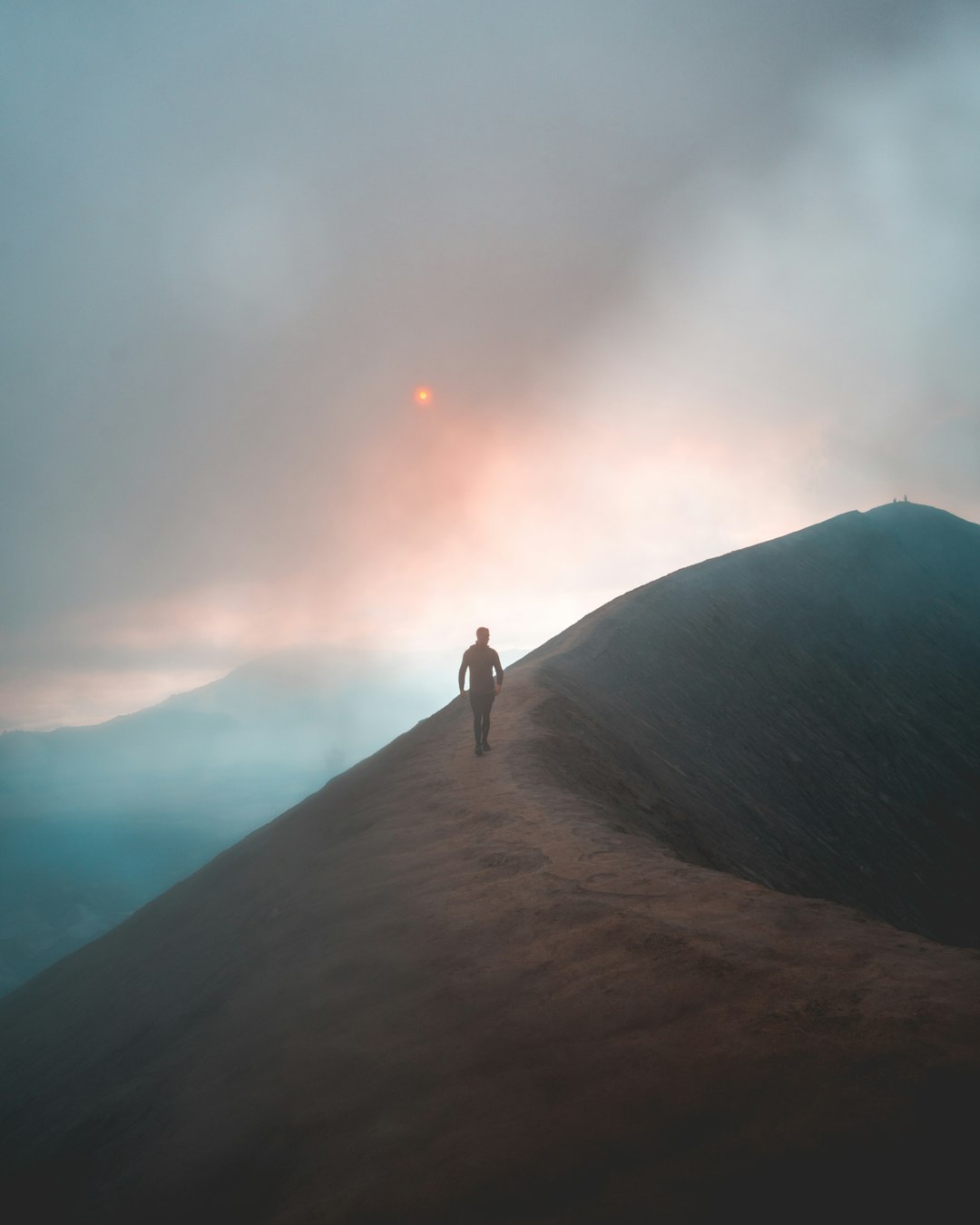
FAQ
- Q: Can I replace return with System.out.print?
A: No, System.out.print only displays values but does not pass them back in code logic, while return does. - Q: Can a method contain both return and System.out.print?
A: Yes, a method can print values and still return a result to the caller. This is common during debugging. - Q: What happens if there’s code after a return statement?
A: Any code after a return in the same block will not be executed, as return exits the method immediately. - Q: Does System.out.print return anything?
A: No, it performs an output action but doesn’t affect program flow or data passing. - Q: Is return required in all methods?
A: No, only methods that declare a return type (like int, String, etc.) need a return. void methods do not.